JavaScript Best Practices for Beginners to Advanced Developers
Ready to take your JavaScript skills to the next level? Explore our latest tutorial, “JavaScript Best Practices” designed for both beginners and advanced developers. Discover coding tips, best practices, and real-world examples that will enhance your code readability and efficiency. Elevate your coding game and become a JavaScript expert today!
These best practices will not only help you write cleaner and more maintainable code but will also contribute to your growth as a JavaScript developer.
Your contributions help me create free premium courses. Buy me a coffee to say thanks and fuel my next project!
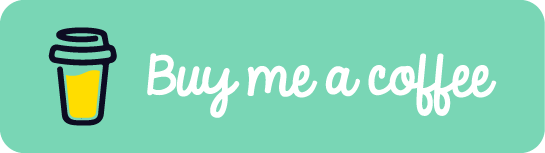
JS Best Practices
Let’s delve into each of the JavaScript best practices, explaining them with examples:
1. Use Meaningful Variable and Function Names:
Always choose descriptive names for variables and functions. This improves code readability and makes maintenance easier.
// Bad
let a = 10;
function x() { /* ... */ }
// Good
let itemCount = 10;
function calculateTotal() { /* ... */ }
2. Understand Variable Scoping:
Understand the difference between var
, let
, and const
. Prefer let
and const
over var
for block-scoped variables.
// Bad
var x = 5;
// Good
let y = 10;
const z = 15;
3. Avoid Global Variables:
Minimize the use of global variables to prevent unintended side effects and conflicts.
// Bad
let globalVar = 42;
// Good
function exampleFunction() {
let localVar = 42;
// use localVar
}
4. Use Strict Mode:
Enable strict mode to catch common coding errors and prevent the use of certain “bad” features.
'use strict';
5. Consistent Code Formatting:
Maintain a consistent code style throughout your project. Consider using tools like ESLint to enforce coding standards.
6. Always Declare Variables:
Avoid using variables without declaring them. This helps catch accidental global variable creations.
// Bad
undeclaredVar = 42;
// Good
let declaredVar = 42;
7. Avoid Using eval():
The eval()
function can introduce security risks and is generally considered bad practice.
8. Prefer === over ==:
Use strict equality (===
) to avoid type coercion issues.
// Bad
if (value == 42) { /* ... */ }
// Good
if (value === 42) { /* ... */ }
9. Handle Errors Gracefully:
Always include error handling in your code to prevent crashes and improve user experience.
try {
// code that might throw an error
} catch (error) {
// handle the error
}
10. Avoid Using Global this:
In functions and callback functions, use bind
or arrow functions to maintain the correct value of this
.
// Bad
function exampleFunction() {
console.log(this);
}
// Good
function exampleFunction() {
console.log(this);
}.bind(this);
// Better
const exampleFunction = () => {
console.log(this);
};
11. Use Template Literals for Strings:
Template literals make string concatenation more readable.
// Bad
let greeting = 'Hello, ' + name + '!';
// Good
let greeting = `Hello, ${name}!`;
12. Avoid Hardcoding Values:
Avoid hardcoding values that might change in the future. Use constants or configuration objects.
// Bad
function calculateTax(income) {
return income * 0.2;
}
// Good
const TAX_RATE = 0.2;
function calculateTax(income) {
return income * TAX_RATE;
}
13. Destructuring Assignment:
Use destructuring assignment for arrays and objects to make code more concise.
// Bad
let firstName = person.firstName;
let lastName = person.lastName;
// Good
let { firstName, lastName } = person;
14. Avoid Using Magic Numbers:
Replace magic numbers with named constants to improve code readability.
// Bad
function calculateArea(radius) {
return 3.14 * radius * radius;
}
// Good
const PI = 3.14;
function calculateArea(radius) {
return PI * radius * radius;
}
15. Use Map, Filter, and Reduce:
Utilize functional programming concepts for cleaner and more concise code.
// Bad
let squaredNumbers = [];
for (let i = 0; i < numbers.length; i++) {
squaredNumbers.push(numbers[i] * numbers[i]);
}
// Good
let squaredNumbers = numbers.map(num => num * num);
16. Avoid Callback Hell (Promises, Async/Await):
Use Promises or Async/Await to handle asynchronous code and avoid callback hell.
// Callback Hell
fetchData(url, data => {
process(data, result => {
display(result, () => {
// more nested callbacks...
});
});
});
// Using Promises
fetchData(url)
.then(data => process(data))
.then(result => display(result))
.catch(error => console.error(error));
// Using Async/Await
async function fetchDataAndProcess() {
try {
const data = await fetchData(url);
const result = await process(data);
display(result);
} catch (error) {
console.error(error);
}
}
17. Modularize Code:
Break down large pieces of code into smaller, reusable modules.
18. Use ES6+ Features:
Familiarize yourself with and use features introduced in ECMAScript 6 (ES6) and later versions.
19. Test Code Regularly:
Implement testing practices, such as unit testing, to ensure code reliability.
20. Keep Learning:
JavaScript is constantly evolving. Stay updated with the latest language features, tools, and best practices.
Your contributions help me create free premium courses. Buy me a coffee to say thanks and fuel my next project!
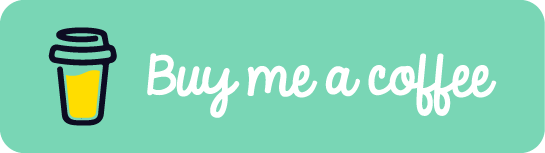